How to Deploy Your Next.js Template in 10 Minutes on Your VPS
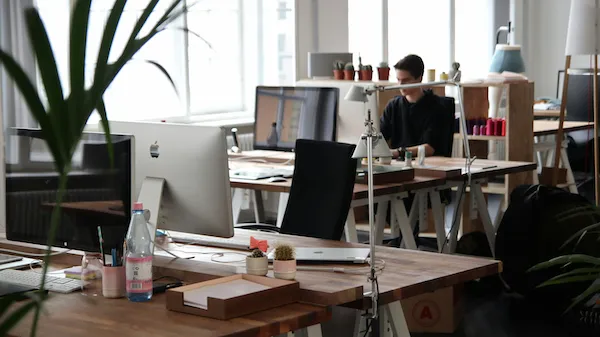
I’ve deployed dozens of Next.js apps over the years, and I can tell you this: you don’t need to overcomplicate it. With a Virtual Private Server (VPS) like DigitalOcean, Linode, or AWS Lightsail, you can go from code to production in under 10 minutes. Let me walk you through the process—no fancy DevOps tools required!
What You’ll Need
Before we start, make sure you have:
A Next.js template (your project folder).
A VPS (I’ll use Ubuntu 22.04 for this guide).
SSH access to your VPS (username + IP/password).
A domain name (optional, but recommended).
Step 1: Connect to Your VPS via SSH
Open your terminal and log in:
bash
Copy
ssh username@your_server_ip
Replace username
and your_server_ip
with your VPS credentials.
Step 2: Install Node.js and npm
Next.js runs on Node.js. Let’s install the latest LTS version:
bash
Copy
curl -fsSL https://deb.nodesource.com/setup_lts.x | sudo -E bash -
sudo apt-get install -y nodejs
Verify the installation:
bash
Copy
node -v # Should show v20.x or higher
npm -v # Should show 10.x or higher
Step 3: Upload Your Next.js Template
Option A: Clone from GitHub
If your template is on GitHub:
bash
Copy
git clone https://github.com/your-username/your-nextjs-template.git
cd your-nextjs-template
Option B: Upload via SFTP
Use tools like FileZilla or Cyberduck to drag-and-drop your template folder to the VPS.
Step 4: Install Dependencies
Navigate to your project folder and run:
bash
Copy
npm install
This installs all packages listed in your package.json
.
Step 5: Build Your Next.js Project
Generate the production build:
bash
Copy
npm run build
This creates an optimized .next
folder with static assets and server-side code.
Step 6: Run the Production Server
Start your app using Next.js’s built-in server:
bash
Copy
npm start
Your app will run on http://localhost:3000
.
But wait! If you close your SSH session, the app will stop. Let’s fix that.
Step 7: Keep the App Running Forever (PM2)
Install PM2, a process manager for Node.js:
bash
Copy
sudo npm install -g pm2
Start your app with PM2:
bash
Copy
pm2 start npm --name "next-app" -- start
Save the process list so it restarts on reboot:
bash
Copy
pm2 save
pm2 startup
Step 8: Set Up a Reverse Proxy (Nginx)
Your app is running, but it’s stuck on port 3000. Let’s expose it to the internet securely.
Install Nginx:
bash
Copy
sudo apt install nginx
Create a Config File:
bash
Copy
sudo nano /etc/nginx/sites-available/nextjs-app
Paste this configuration (replace your_domain.com
with your actual domain or IP):
nginx
Copy
server {
listen 80;
server_name your_domain.com;
location / {
proxy_pass http://localhost:3000;
proxy_http_version 1.1;
proxy_set_header Upgrade $http_upgrade;
proxy_set_header Connection 'upgrade';
proxy_set_header Host $host;
proxy_cache_bypass $http_upgrade;
}
}
Save with Ctrl+X
→ Y
→ Enter
.
Enable the Configuration:
bash
Copy
sudo ln -s /etc/nginx/sites-available/nextjs-app /etc/nginx/sites-enabled/
sudo nginx -t # Test for errors
sudo systemctl restart nginx
Step 9: Set Up SSL (Optional but Recommended)
Secure your site with Let’s Encrypt:
bash
Copy
sudo apt install certbot python3-certbot-nginx
sudo certbot --nginx -d your_domain.com
Follow the prompts, and HTTPS will auto-configure!
Step 10: You’re Live!
Visit http://your_server_ip
or https://your_domain.com
in your browser. Your Next.js template is now live on your VPS!
Troubleshooting Tips
App not loading? Check PM2 logs:
bash
Copy
pm2 logs next-app
Nginx issues? Test your config:
bash
Copy
sudo nginx -t
Port blocked? Allow traffic through Ubuntu’s firewall:
bash
Copy
sudo ufw allow 'Nginx Full'
Why This Works
PM2 ensures your app survives crashes or reboots.
Nginx handles traffic efficiently and adds security layers.
VPS gives you full control without shared hosting limitations.
Next Steps
Connect a custom domain (if you haven’t already).
Automate deployments with GitHub Actions or Git hooks.
Monitor performance using PM2’s built-in tools:
bash
Copy
pm2 monit
Deploying Next.js on a VPS isn’t just fast—it’s empowering. You own the entire stack, from server to code. Once you’ve done this a few times, you’ll wonder why anyone pays for expensive hosting platforms. Happy deploying! 🚀
Need help? Drop your VPS provider name in the comments, and I’ll share specific optimizations!